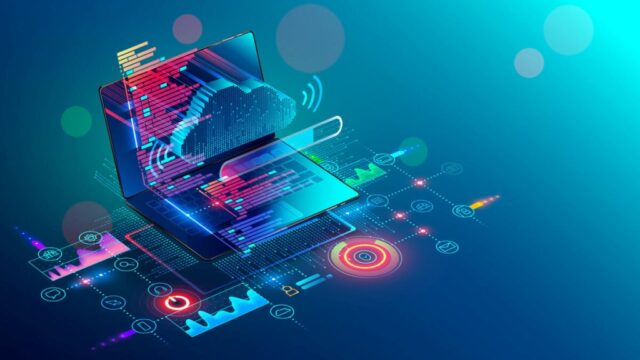
Java is a programming language that is cross-platform (runs on any computer that has a Java Virtual Machine), and allows you to create desktop applications using a scripting language. Java is an object-oriented language which allows you to create powerful applications (desktop, server, etc), and is used by a lot of companies . It is one of the only programming languages that is written in a free and open source environment (the other being Python, which is also open source) and is one of the most used. Java Machine Learning Projects in depth, A book by Kishore B.M.G. Thesis, Published by Springer India. This document provides an overview of the basic concepts of Java and programming.
Structure of Java classes
A Java class is described in a text file with the extension .java. In the example below, the Java keywords are in bold. package [package_name]; import [other_packages] ; public class name { [variables (also called fields)]; [constructor(s)]; [other methods]; }
- The keyword package defines the position of a particular class relative to other classes and provides a level of access control. Access modifiers (such as public and private) will be used later in this tutorial.
- The import keyword defines other classes or class groups you use in your class. The import statement is used to indicate what the compiler should look for when resolving the class names used in a given class.
- The keyword class is placed before the name of this class. The class name and file name must match if the class is declared public (which is good practice). However, the public keyword before the class keyword is a modifier and is optional.
- Variables or data attached to programs (such as integers, strings, arrays, and references to other objects) are called instance fields (often abbreviated to fields).
- Constructors are functions that are called when creating (instantiating) an object (the memory representation of a Java class).
- Methods are functions that can be executed on an object. They are also called instance methods.
Single class
To execute a program in Java, you must define the main method in the Java class, as in the following example. The main method is automatically called when the class is called from the command line. public class Simple { public static void main(String args[]){ } } The command line arguments are passed to the program via the array args[]. Message: A method modified with the static keyword is called without reference to a specific object. Instead, the name of the class is used. These methods are called class methods. The main method is a special method that is called when this class is executed with the Java runtime environment.
Naming conventions in Java
- Class names must be written in capital letters, with the first letter being a capital letter and the first letter of each internal word being a capital letter. This approach is called the upper camel register.
- Methods must be capitalized verbs: the first letter is lower case, and the first letter of each internal word is capitalized. This is called a lower camel register.
- Names of variables should be short but meaningful. The choice of variable name must be meaningful: It should indicate to a casual observer the purpose of the use.
- Variable names of one character should be avoided, except for temporary variables in Throwaway.
- Constants must be declared with capital letters.
Message: The last keyword is used to indicate a variable whose value can only be assigned once. Once an end variable is assigned, it always contains the same value.
Compile and run
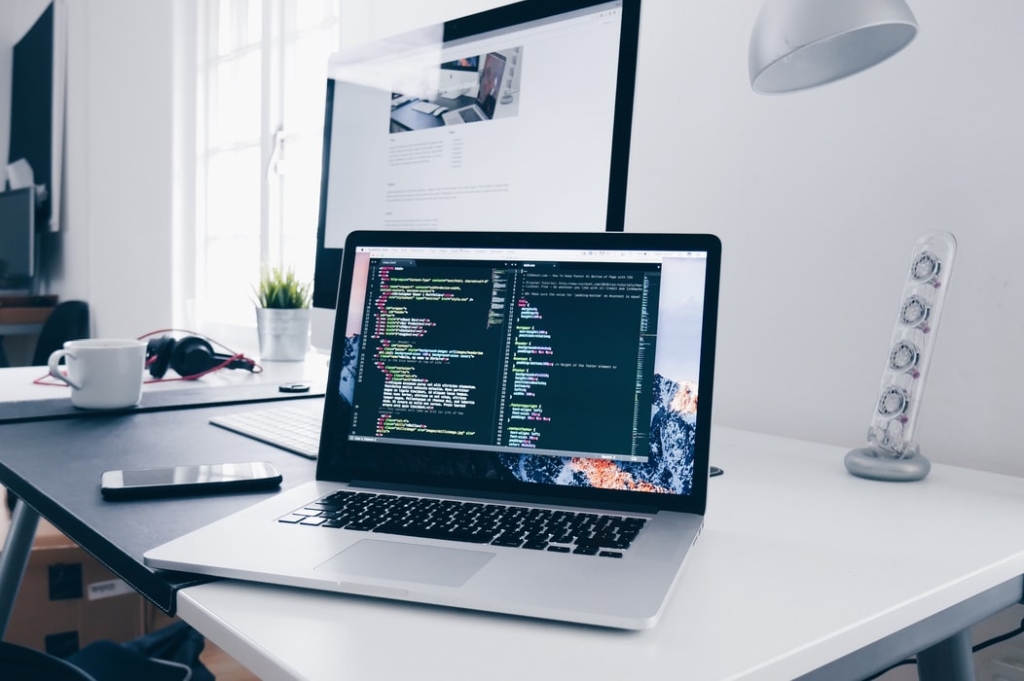
Java class files must be compiled before they can be executed. To compile a Java source file, use the Java compiler (javac). javac -cp [path to other classes] -d [compiler output path] [source path].java You can use the CLASSPATH environment variable to specify the directory above the location of the package hierarchy. After compiling the .java source file, a .class file is created. To run a Java application, start it with the Java interpreter (java) : java -cp [path to other classes] [package name].
CLASS PATH
The default value for classpath is the current working directory (.); however, specifying the CLASSPATH variable or the -cp command line option overrides this value. The CLASSPATH variable is used by both the Java compiler and the Java interpreter (runtime). The classpath can :
- A list of directory names (separated by semicolons under Windows and colons under UNIX) – the classes are located in the package structure relative to one of the directories in the list.
- A .zip or .jar file name that is completely path-determined – the classes in these files should be archived with path names derived from the directories formed by their package names.
Message: The directory containing the root of the package tree must be added to the classpath. Consider putting the classpath information in a command window or even in a Java command, rather than hard coding it into the environment.
Compile and run: Example
Consider the following simple class in a file named HelloWorld.java in the test directory of the path /home/oracle : public class HelloWorld public static void main (String [] args) { System.out.println( Hello World); } } Compile the HelloWorld.java program with the following command: To run the application, use an interpreter and a class name : java Hello World Hello World The advantage of using an IDE like NetBeans is that classpath management, compilation and execution of a Java application are all done with this tool.
Units of code
Java fields (variables) and methods have a class range defined by a left brace ending with a right brace closing. The scope of the class allows any method of the class to call or invoke any other method of the class. The scope of the class also allows any method to access any field in the class. For example: public class SayHello { public static void main(String[] args) { System.out.println(Hello World); } } Code blocks are always defined by square brackets {}. The block is executed by executing each of the instructions defined in the block in order from beginning to end (left to right). The Java compiler removes unnecessary white space in a line of code. Line breaks are not necessary, but they make the code more readable. In this post, the line indentation is four spaces, which is the default indentation used in NetBeans IDE.
Primitive data types
Whole
Java provides four different types of integers to handle numbers of different sizes. All numeric types are signed types, meaning they can store positive or negative numbers. The integer types have the following ranges:
- The byte range is -128 to +127. Number of bits = 8.
- short range from -32.768 to +32.767. Number of bits = 16.
- int between -2 147 483 648 and +2 147 483 647. The most common integer type is int. Number of bits = 32.
- long range of -9,223,372,036 854,775,808 to +9,223,372,036 854,775,807. Number of bits = 64.
Floating point
Floating point types store numbers with a decimal part and comply with the IEEE 754 standard. There are two types of slip points: Float and double. The term double is so named because it offers double precision compared to float. 32 bits are used to store data in float format, while 64 bits are used in double format.
Numeric characters
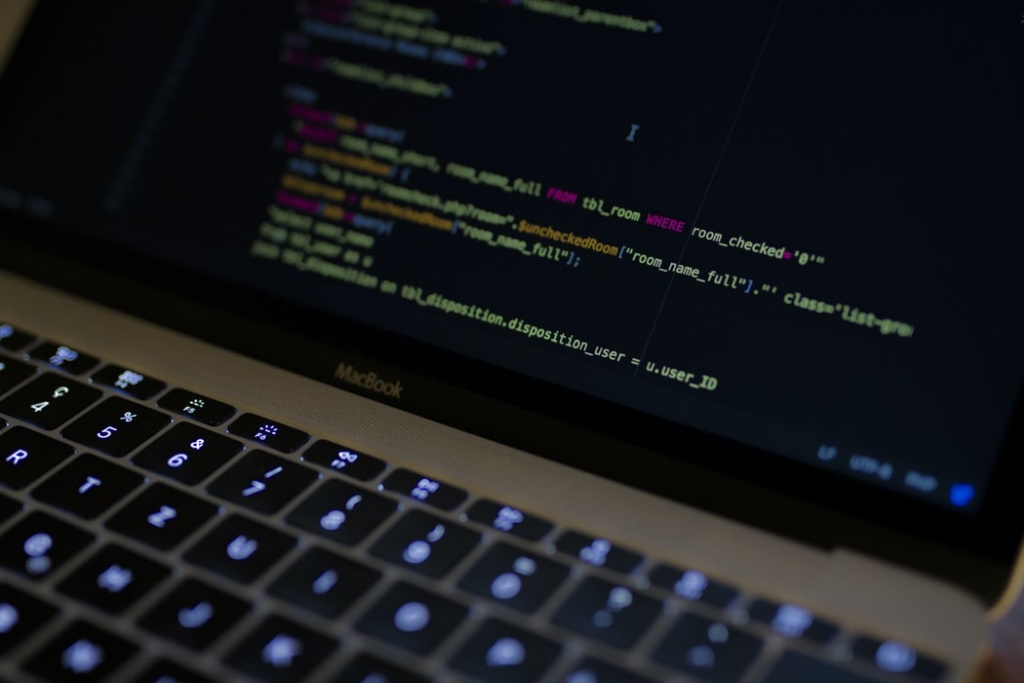
Any number of underscores (_) can be placed between the digits of the numeric field. This can improve the readability of your code. lang creditCardNumber = 1234_5678_9012_3456L; lang socialSecurityNumber = 999_99_9999L; lang hexBytes = 0xFF_EC_DE_5E; lang hexWords = 0xCAFE_BABE ; lang maxLong = 0x7fff_ffffff_ffffffL; byte nybbles = 0b0010_0101; lang bytes = 0b11010010_01101001_10010100_10010010010 ;
Rules for characters
Underscores can only be placed between numbers; underscores cannot be placed in the following positions:
- At the beginning or at the end of the song
- Connection to the decimal point in a floating point
- To get the suffix F or L
- In items where a sequence of numbers is expected
Message: The Java language is case sensitive. In Java, the variable creditCardNumber is different from CREDITCARDNUMBER. By convention, Java variable and method names use lower case – lower case for the first letter of the first element of a variable name, and upper case for the first letters of subsequent elements.
Operator
Simple assignment operator
= Simple assignment operator
Arithmetic operators
+ addition operator (also used to link sequences) – subtraction operator * multiplication operator / division operator % residual operator
Unary operators
+ + unary operator; returns a positive value – Min unary operator; negates the expression ++ Increment operator; increases the value by — Decrement operator; decreases the value by ! Boolean complement operator; reverses the value of a Boolean value. As numbers are entered, see the section above for a list of commonly used operators. Most are common to all programming languages, and a description is provided for each. Binary and bitwise operators are omitted for brevity. Message: The operators have the last priority. The priority can be replaced by the use of parentheses.
Logic operators
Equality and kinship operators
== equal to != not equal to >= greater than >= greater than or equal to < smaller than <= less than or equal to
Conditional instructions
Condition-EN ||Condition-OR ? Ternary (short for if-then-else)
Type of comparator
instanceof Compares an object with the specified type.
if different Instruction
The following example illustrates the syntax of the if-else statement in Java. 1 public class IfElse { 2 3 public static void main(String args[]){ 4 long a = 1; 5 long b = 2; 6 7 if (a == b){ 8 System.out.println(True); 9 } else { 10 System.out.println(False); 11 } 12 13 } 14 } The else statement provides a secondary execution path if the if statement fails on false. The result of the above code is False.
Application of switch
The switch operator is used to compare the value of a variable with different values. For each of these values, you can define a set of operators to execute. The switch operator evaluates its expression and then executes all operators that follow the corresponding case label. Each interrupt operator terminates the switch operator it contains. The interrupt operators are needed because without them, the circuit block operators are not executed: All operators following the corresponding case label are executed sequentially, regardless of the expression of the following case labels, until a break operator occurs. 1 public class SwitchStringStatement { 2 public static void main(String args[]){ 3 4 String color = Blue; 5 String shirt = Shirt; 6 7 switch (color){ 8 case Blue: 9 shirt = blue + shirt; 10 pause; 11 case Red: 12 shirt = red + shirt; 13 pause; 14 default: 15 shirt = white + shirt; 16 } 17 18 System.out.println(shirt-type: + shirt); 19 } 20 } Message: The decision to use the if-then-else statement or the switch statement is based on readability and the expression that controls the statement. The if-then-else statement can check expressions based on sets of values or conditions, while the switch statement only checks expressions based on a single integer, character, enumerated value, or string object. In Java SE 7 and later, you can use a String object in the switch operator expression. The above code snippet illustrates the use of strings in the switch operator.
, while loop
The while loop performs a check and continues when the expression evaluates to true. package com.example.review; public class WhileTest { public static void main(String args[]) { int x = 10; while (x < 20) { System.out.print(value of x: + x); x++; System.out.print(n); } } } The while operator evaluates an expression to produce a boolean value. If the expression is true, the while statement executes the statements in the while block. The while loop above cycles through the array using a counter. Conclusion from the above example: Value x : 10 x-value : 11 x-value : 12 x-value : 13 x-value : 14 x-value : 15 x-value : 16 x-value : 17 x-value : 18 x-value : 19 There is also a do-while loop where the test is run at least once after the expression. The difference between do-while and while is that do-while evaluates the expression at the end of the loop, not at the beginning.
for loop
The for statement is a compact way to iterate over a set of values – the loop is repeated until a certain condition is met. 1 public class ForLoop { 2 3 public static void main(String args[]){ 4 5 for (int i = 0; i < 9; i++ ){ 6 System.out.println(i : + i); 7 } 8 9 } 10 } The counter is initialized and incremented for each step of the for loop shown in the example above. If the condition operator returns false (if i is no longer less than 9), the loop is interrupted. Here is an example of the output of this program. i : 0 i : i : 2 i : 3 i : 4 i : 5 i : 6 i : 7 i : 8
Arrays and the for-each loop
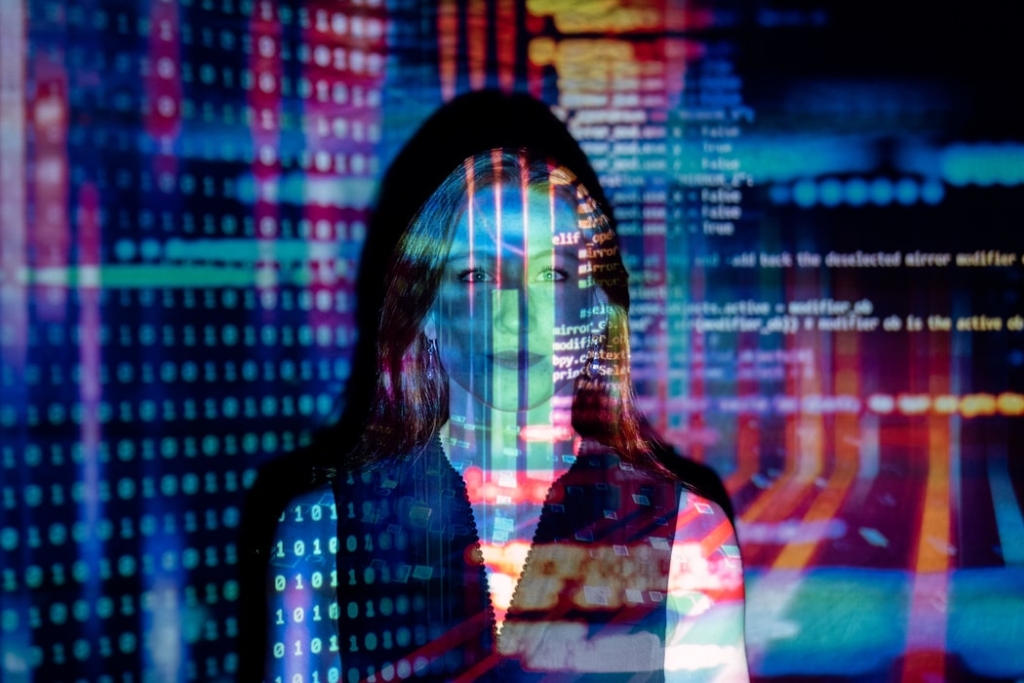
This course shows how to define arrays in Java and how to iterate over arrays using the foreach loop. 1 public class ArrayOperations { 2 public static void main(String args[]){ 3 4 String[] names = new String[3]; 5 6 names[0] = Blue shirt; 7 names[1] = Red shirt; 8 names[2] = Black shirt; 9 10 int[] numbers = {100, 200, 300}; 11 12 for (String name:names){ 13 System. 14 } 15 16 for (int number:numbers){ 17 System.out.println(Number : + number); 18 } 19 } 20 } The first array, names, creates an array of strings and initializes each element separately. The second matrix, numbers, is a matrix of integers. Each array is passed through with the Java for-each construction. The enhanced for-loop is used to make the for-loop more compact and organized. It is used to access each subsequent value in the value set. It is typically used to iterate over an array or a collection class (such as Array or ArrayList): Name: Blue Shirt Name : Red Shirt Name : Black shirt number : 100 Number : 200 Number : 300 Message: Arrays are also standard objects. All arrays support the methods of the Object class, so you can always determine the size of an array by its length field.
strings
The following code shows how text characters are displayed in Java. 1 public class Strings { 2 3 public static void main(String args[]){ 4 5 char letter=a; 6 7 String string1 = Hello; 8 String string2 = World; 9 String string3 = ; 10 String dontDoThis = new String (Bad Practice); 11 12 string3 = string1 + string2; // concatenation of strings 13 14 System.out.println(output: + string3 + + letter); 15 16 } 17 } Individual characters can be displayed with the char type. However, Java also has a string type to display multiple characters. Texts can be defined as shown above and concatenated using the + sign as the concatenation operator. The code on the slide gives the following result: Warning: The class String is immutable: Once created, the contents of an object of the String class can never be changed. The immutability of chain objects helps the JVM to reuse chain objects, which reduces memory usage and improves performance. Texts should always be initialized with the = assignment operator and the text in quotes, as in the examples. It is strongly discouraged to use new to initialize strings. The reason is that Bad Practice in line 10 is a string literal. The keyword new simply creates another instance that is functionally identical to the literal one. If this statement were to occur in a frequently called loop, many unnecessary string instances could be created.
String operations: StringBuilder
The following example illustrates the use of the StringBufilder class and the most commonly used methods. public class StringOperations { public static void main(String arg[]) { StringBuilder sb = new StringBuilder(hello); System.out.println(string sb : + sb); sb.append( world); System.out.println(string sb : + sb) ; sb.append( !).append( are).append( u ?); System.out.println(string sb : + sb) ; iem.insert(12, How); System.out.println(string sb : + iem) ; // Length receive System.out.println(length: + sb.length()) ; // Received sub stringSystem.out.println(Sub: + sb.substring(0, 5));}
StringBuilder class
StringBuilder objects are similar to String objects, except that they are mutable. The StringBuilder class is used when working with large strings or when the content of a string is changed frequently. The above example illustrates modifying a string with the append and insert methods, and concatenating with the append method.
Output:
sb: hello sb: hello world sb: hello world! is that you? sb: hello world! How does it work? Length: 25 Sub : Hello
Simple Java Class Employees
A Java class is often used to store or display data for a construct representing the class. For example, you can create a template (program view) of an employee. The Employee object defined with this template contains values for empId, name, social security number (ssn), and salary. 1 package com.example.domain; 2 public class Employee { 3 public int empId; 4 public String name; 5 public String ssn; 6 public double salary; 7 8 public Employee () { 9 } 10 11 public int getEmpId () { 12 return empId; 13 } 14 } The constructor is used to create an instance of the class. Unlike methods, constructors do not declare a return type and are declared with the same name as their class. Constructors can take arguments, and you can declare more than one constructor.
Methods
When a class has data fields, it is common to provide methods to store data (setter methods) and retrieve data (getter methods) from the fields. 1 package com.example.domain; 2 public class Employee { 3 public int empId; 4 // other fields… 5 public void setEmpId(int empId) { 6 this.empId = empId; 7 } 8 public int getEmpId() { 9 return empId; 10 } 11 // getter/setter methods for other fields… 12 }
Add instance methods to the class Employee
It is common to create a set of methods that can be used to store the data in the : Methods that set the value of each field, and methods that retrieve the value of each field. These methods are called getters and setters. It is common to use set and get plus the field name, with the first letter of the field name in upper (lower) case. Most modern integrated development environments (IDEs) provide an easy way to automatically generate getter and setter methods for you. Note that the set methods use the this keyword. The keyword this allows the compiler to distinguish the name of the class field (this) from the name of the parameter passed as an argument. Without the keyword, the net effect is that you assign a value to yourself. (In fact, NetBeans gives a warning: auto-task). In this simple example, you can use the setName method to change an employee’s name and the setSalary method to change an employee’s salary. Message: The methods declared on this slide are called instance methods. You are called with an instance of this class.
Creating an instance of class
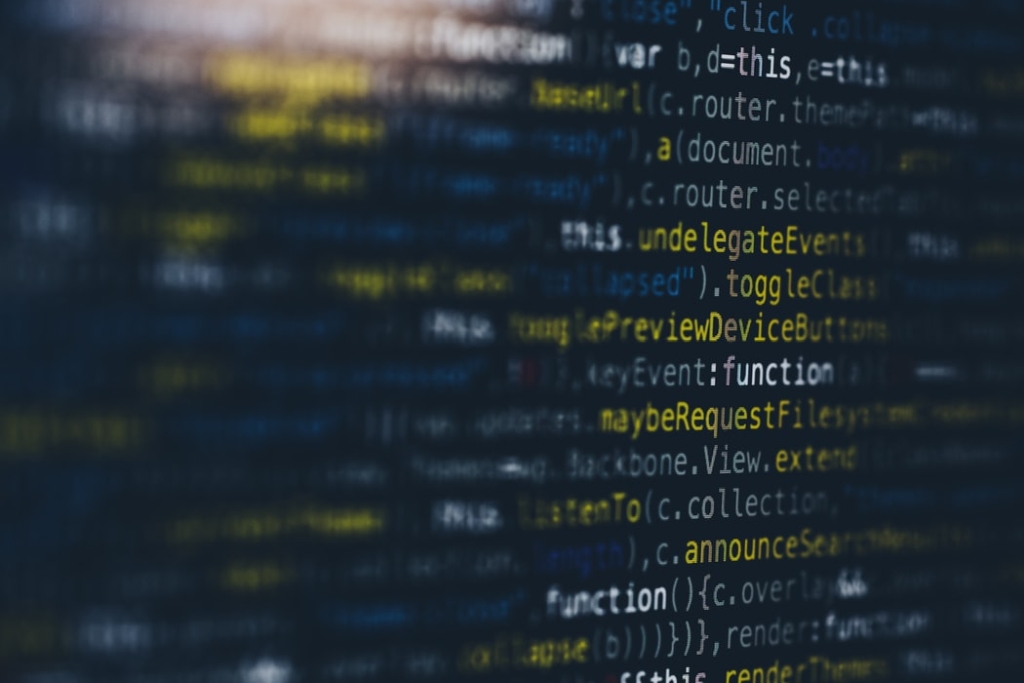
To build or create an instance (object) of the Employee class, use the new keyword. /* In another class or main method */ Employee emp = new Employee(); emp.empId = 101; // legal if the field is public, // but not good practice OO emp.setEmpId(101); // use method emp.setName(John Smith) instead; emp.setSsn(011-22-3467); emp.setSalary(120345,27);
Create an instance of the class Employee
- You must allocate memory for the Employee object and call the class constructor to initialize all variables in the class instance.
- The new operator is used for memory allocation, and the constructor is called to initialize all variables of the instance of the class.
- An object instance is created when you use the keyword new with the constructor. All fields declared in the class are given space in memory and are initialized with default values.
- If the memory allocation and constructor were successful, a reference to the object is returned as the result. In the above example, the reference is assigned to a variable named emp.
- After setting the values for all data fields, you have an employee instance with an empId value of 101, a name with a string of John Smith, a social security number (ssn) string set to 011-22-3467, and a salary with a value of 120,345.27.
Design Engineers
The constructor is used to create an instance of the class. Builders can accept parameters. The constructor is declared with the same name as the class. public class Employee { public Employee() { } } Employee emp = New Employee() ;
Manufacturers of standard and non-standard products
- Every class must have at least one constructor.
- If you don’t specify them in the class declaration, the compiler creates a default constructor that takes no arguments when called.
- The default constructor initializes instance variables to the initial values specified in their declarations or to the default values (null for primitive numeric types, false for boolean values, and null for references).
- If you declare class constructors, the compiler will not create a default constructor.
- In this case, you should declare a constructor without arguments if default initialization is required.
- Like the default constructor, the constructor is called without arguments with empty brackets
Application of the package
The keyword package is used in Java to group classes. A package is implemented as a directory and, like a directory, provides a namespace for a class.
Packaging
In Java, a package is a group of types (classes). There can be only one package declaration for a file. Packages form a namespace, a logical collection of things, similar to a directory hierarchy. Packages avoid name conflicts and also provide access control to classes. A good habit is to always use the package declaration.
Import request
In your applications, you can refer to a class by its full namespace, as in the following example: java.util.Date = new java.util.Date() ; But for that you have to type a lot of things. Instead, Java provides an import operator that you can use to indicate that you want to refer to a class from another package. Notes: A good practice is to use a specific, fully qualified package and class name to avoid confusion when two classes have the same name, as in the following example: java.sql.Date and java.util.Date. The first is a date class used to store the date type in the database, and java.util.Date is a general purpose date class. It turns out that java.sql.Date is a subclass of java.util.Date. Modern IDEs like NetBeans and Eclipse automatically search for import operators and add them for you. In NetBeans, for example, B. use the key combination Ctrl + Shift + I to correct imports in your code.
Summary
The import keyword is used to identify the classes you want to refer to in your class. The import operator provides a convenient way to identify the classes you want to refer to in your class. You can import a single class or an entire package: You can include multiple input operators: import java.util.Date; import java.util.Calendar ; It is recommended to use the full package and class name and not the wildcard * to avoid conflicts with class names.
Import request
The import operators follow the package declaration and precede the class declaration. An import declaration is not required. By default, your class always imports java.lang.*. It is not necessary to import classes that are in the same package: package com.example.domain; import com.example.domain.Manager; // import unused
Java is a master key
The Java language uses value passing for all assignment operations. This means that the argument to the right of the equal sign is evaluated and the value of the argument is assigned to the left of the equal sign. To represent this with primitives, consider the following: The value of x is copied and transferred to y. If x changes (e.g. x = 5 ;), the value of y remains unchanged. For Java primitives, it’s easy. Java does not pass a reference to a primitive (such as an integer), but rather a copy of the value.
Transfer by value for object references
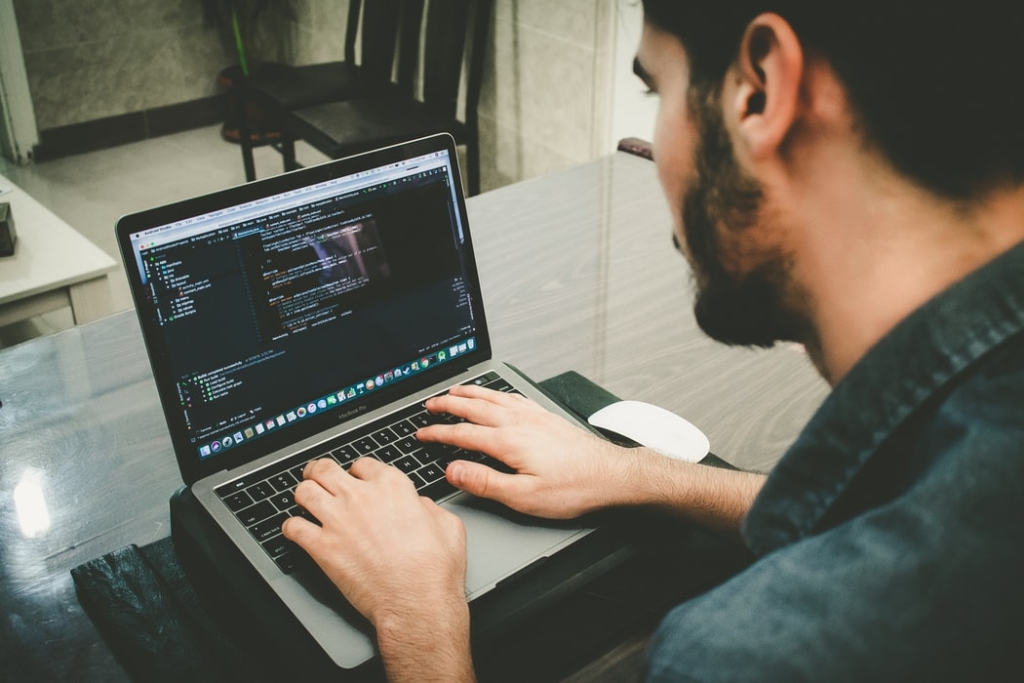
For Java objects, the value on the right side of the assignment is a reference to the memory where the Java object is stored. Employee x = New employee(); Employee y = x ; A reference is an address in memory. If you assign the value x to the value y, you do not create a new employee object, but a copy of the reference value. Message: An object is an instance of a class or an array. Reference values (references) are references to these objects, as well as a special null reference that points to no object.
Objects transmitted as parameters
4 public class ObjectPassTest { 5 public static void main(String[] args) { 6 ObjectPassTest test = new ObjectPassTest(); 7 Employee x = new Employee (); 8 x.setSalary(120_000.00); 9 test.foo(x); 10 System.out.println (salary employee : 11 + x.getSalary()); 12 } 13 14 public void foo(employee e){ 15 e.setSalary(130_000.00); 16 e = new Employee(); 17 e.setSalary(140_000.00); 18 } In line 6, a new Employee object is created and a reference to this object is assigned to the variable x. In line 8, the pay field is set to 120_000. On line 9, a reference to x is passed to the method foo. Line 15 of method foo sets the value of salary e to 130_000. Since e refers to the same reference as x, the wage value of x is now 130_000. Line 16 creates a new Employee object that modifies the reference stored in e. Now x is not mentioned in the foo method. Line 17 defines the Salary type, but x is not assigned because e no longer contains a reference to x. The program produces the following results: Employee salaries: 130000.0
Refuse collection service
When an object is instantiated with the keyword new, memory is allocated to it. The scope of an object reference depends on where the object is instantiated: public void someMethod() { Employee e = new employee(); // Operations on e } When someMethod ends, the memory referenced by e is no longer available. The Java garbage collector detects when an instance is no longer available and should not be retrieved. The waste is automatically collected by the JVM. The timing of the acquisition depends on many factors.Java is a programming language that you can use to create your very own apps. It’s a very powerful and flexible language, but as with any language, it can get confusing if you don’t know what you’re doing. Java is a bit challenging, but it only takes a little bit of practice to be able to understand and operate it.