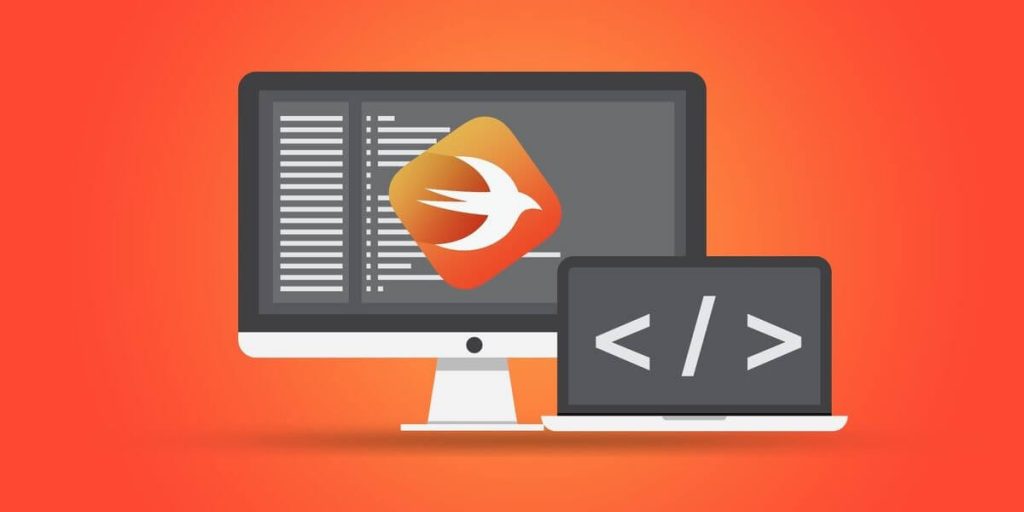
This blog post introduces Swift, a programming language that is being used by Apple. Swift is a programming language developed by Apple that focuses on simplicity and safety. It is also a high-performance programming language, which means that it is designed for building fast, efficient and reliable applications. Swift is the programming language of choice for developing applications for Apple mobile devices including iPhones, iPads and Macs. It is also now the default language for developing applications for Apple’s OS X and tvOS operating systems.
I recently finished a new Swift app called computea ( https://github.com/Ting/computea) and I thought I’d share some of the details of how it was built. Swift is a dynamic language with a focus on performance and safety, and it’s quite interesting to see what kind of application you can build with it. This post is mostly a simple walkthrough of the code, with comments and a few screenshots to give a feel for how the framework works.
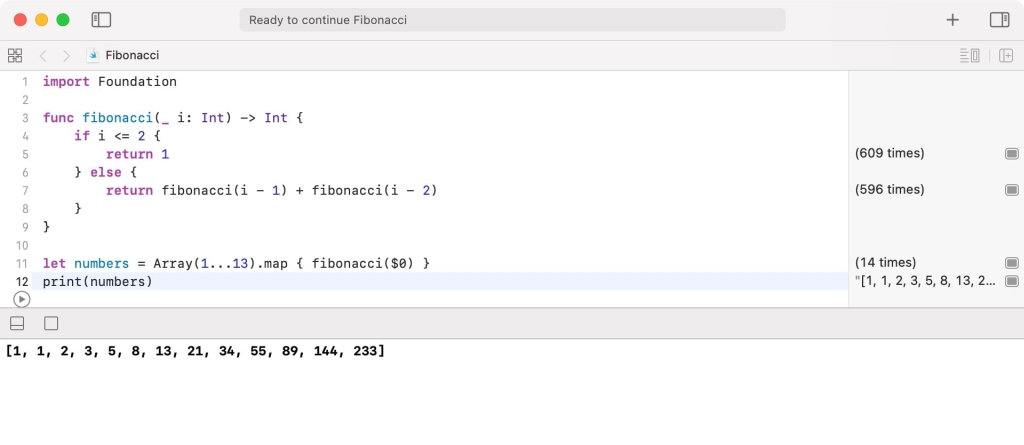
I was looking to learn how to write a computational framework, something that will be easier to read than a traditional language, and something that can run on both iOS and Mac OSX. So, I decided to write my own language, called Swift for Computation. Its primary feature is that it is a mathematical language, meaning that it is capable of running on the CPU, as well as being able to handle mathematical expressions. It is my hope that this post will introduce Swift for Computation.
Euler
Euler is a mathematical framework with many useful mathematical functions. It contains functions for most areas of mathematics, such as algebra, number theory, statistics, etc. It is designed to help you turn your computer into a math guru.
Setting
You can use the following Euler installation options, but we recommend using CocoaPods or SPM.
CocoaPods
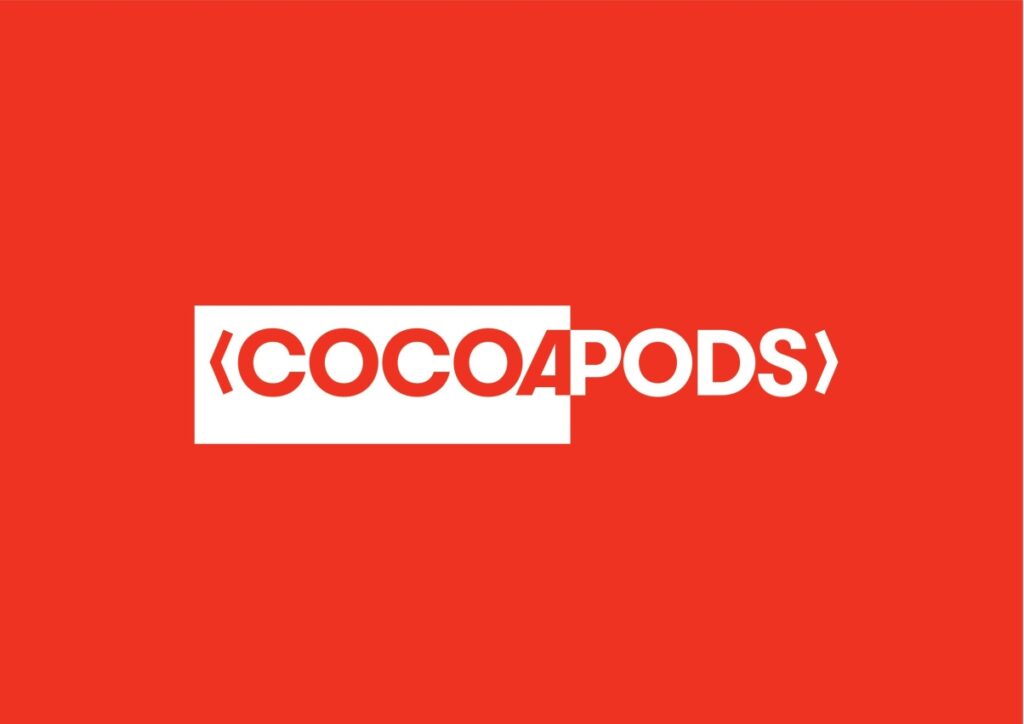
CocoaPods is a dependency manager for Cocoa projects. Instructions for use and installation are available on their website. To integrate Euler into your Xcode project with CocoaPods, specify it in your podfile: pod Euler, ~> 0.3.6.
Carthago
Carthage is a decentralized dependency manager that collects your dependencies and provides you with binary frameworks. To integrate Euler into your Xcode project with Carthage, specify it in your cartfile: github arguiot/Euler ~> 0.3.6
Swift package manager
The Swift package manager is a tool integrated with the Swift compiler to automate the distribution of Swift code. It is still in the early stages of development, but Euler supports its use on supported platforms. If the Swift package is installed, add Euler as a dependency to the dependency value in the Package.swift file. Dependencies : [ .package(url : https://github.com/arguiot/Euler.git, .upToNextMajor(from : 0.3.6)) ]
Manual
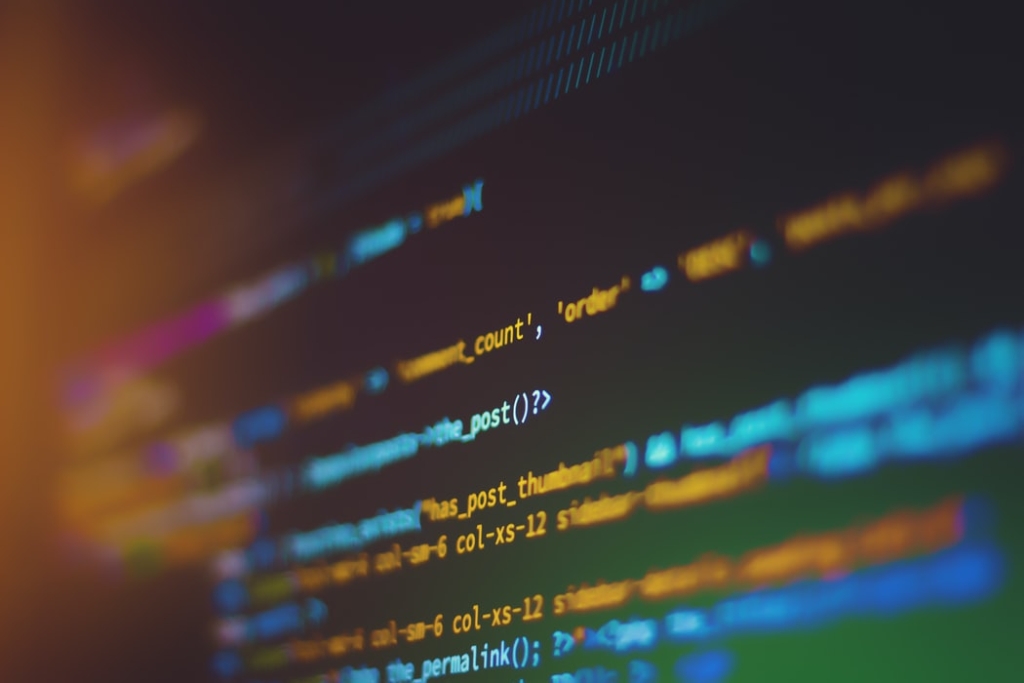
If you don’t want to use one of the above dependency handlers, you can also integrate Euler into your project manually.
Frame
Open Terminal, navigate to the project’s top-level directory, and run the following command if your project is not initialized as a Git repository:
$ git init
Add Euler as a git submodule by running the following command:
$ git submodule add https://github.com/arguiot/Euler.git
Open a new Euler folder and drag and drop Euler.xcodeproj into your application’s Xcode project browser.
It should appear under the blue project icon of your application. Whether it is above or below all other Xcode groups is irrelevant.
- Select Euler.xcodeproj in the project browser and make sure the deployment target matches the target of your application.
- Then select the application project in the Project Browser (blue project icon) to access the Targets configuration window and select the application target under the Targets header in the sidebar.
- In the tab bar at the top of this window, open the General panel.
- Click the + button in the embedded binaries area.
- You will see two different Euler.xcodeproj folders, each containing two different versions of Euler.framework, nested in the Products folder.
It does not matter which product folder you choose, but it is important to know whether you choose the upper or lower Euler.framework. Choose the top Euler.framework for iOS and the bottom framework for macOS. You can check which one you have chosen by looking in your project’s construction log. The build target for Euler is listed as Euler iOS, Euler macOS, Euler tvOS, or Euler watchOS. And that’s it!
Euler.framework is automatically added as target dependency, linked framework and embedded framework during the build phase when the files are copied, which is all that is needed for building on the simulator and device. Here is a list of applications that work with Euler (not exhaustive) Euclid Calculator Euclid is a modern, fully functional calculator for macOS, designed to replace the standard calculator application. Euclid uses Euler to analyze and evaluate all calculations. It leans heavily on the algebra and arrays modules.
Inspiration
The project was strongly inspired by :
Why Swift
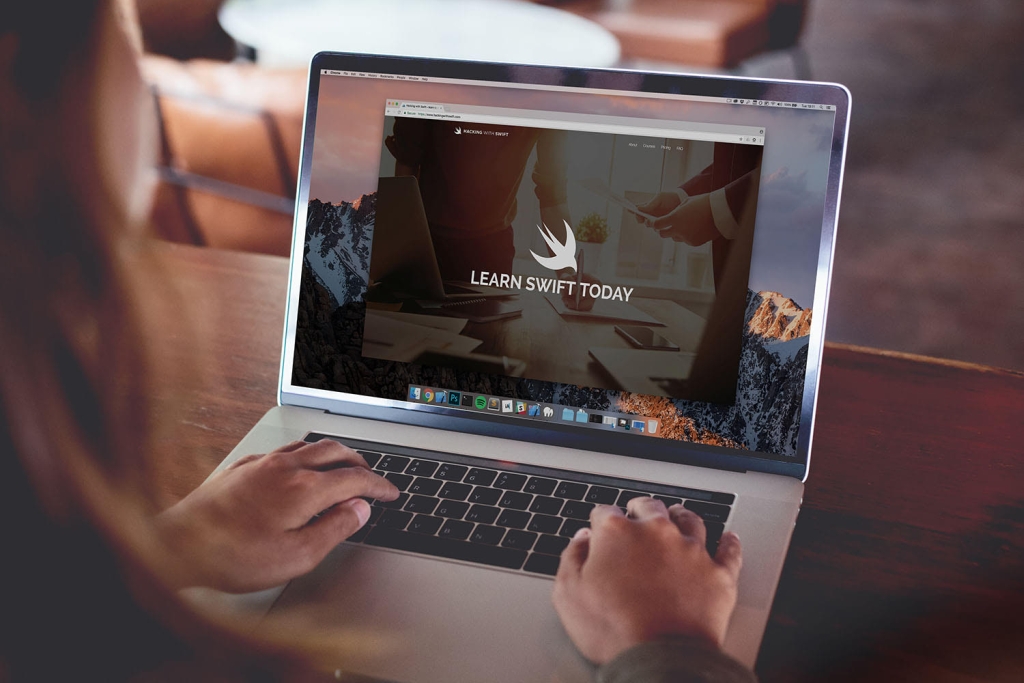
In retrospect, it’s not surprising that Swift is a good fit for the needs of this project. Swift is designed and built by a close-knit team. This team has already developed a highly modular and compileable compiler infrastructure (LLVM), a compiler and runtime for a highly dynamic Smalltalk-derived language (Objective-C), a compiler for a highly static language with an advanced generic system (C++), and a path-sensitive static analysis mechanism (Clang static analyzer). Furthermore, the goal of Swift’s development was to create a language that was as easy to learn and use as a scripting language, but powerful enough to be used as a systems programming language. Swift was the ideal language because of its power, modularity, easy-to-read syntax and parallel API. Swift aims to maximise code clarity and therefore aims to reduce the number of boilerplate codes. The ultimate goal of Swift is to optimize the time it takes to write and maintain a project, including time spent debugging and things other than just writing code.
Target
Euler’s goal is to provide the basic elements of numerical computation in Swift as a set of lightweight modules combined into a single Swift package. Euler was designed to experiment with Swift and its mathematical capabilities. It will also serve as a database for algorithms that can be ported to other languages.
Organisation
Euler is structured around two main areas: BigDouble and BigInt. They are both used to represent large numbers accurately. Euler can be considered as a collection of modules:
GitHub
https://github.com/arguiot/EulerComputation is at the center of our lives. Computers, smartphones, robots, cars, satellites, and even the Internet are all made up of individual components that could be called “computers.” It’s not just the machines we use, but the processes that go into creating them that are computationally intensive.