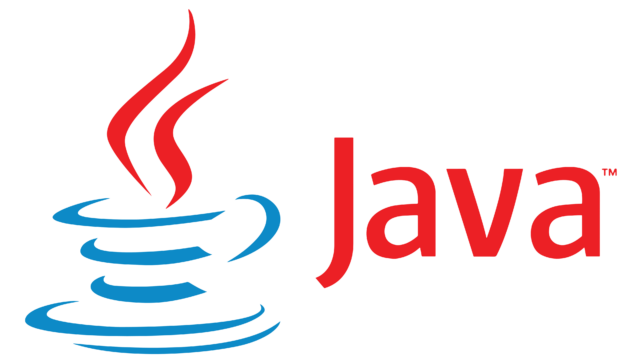
The purpose of this blog is to share the knowledge that I have gathered while working on my projects and also to share my experiences with others. This blog will feature topics related to programming in Java, Android, and iOS.
In this article, we will see how to implement the Java class Sequence Input Stream in Java. It is essentially a wrapper class for a Reader, which can be used to read the content of a sequence of bytes one by one.
Get up to speed with the latest information on Java technology with your free newsletter that will keep you up-to-date on the latest Java technology.
A Sequence Input Stream in Java is a single input stream (file) that is a combination of two or more input streams (files) in a particular order. It reads data from two or more input streams, one after the other. Java Sequence Input Stream starts reading data from a specified set of input streams.
It first reads the data (in bytes) from the first stream in the sequence, then all data from the second stream in the sequence, then all data from the third stream, and so on. When the end of a stream (file) is reached, that file is closed; the next data comes from the next input stream (file). This process continues until the end of the file is reached on the last of the recorded input streams. Therefore, merging two or more files into a single file is called file concatenation and can be done with the Sequence Input Stream class in Java.
Declaration of the Java class Sequence Input Stream
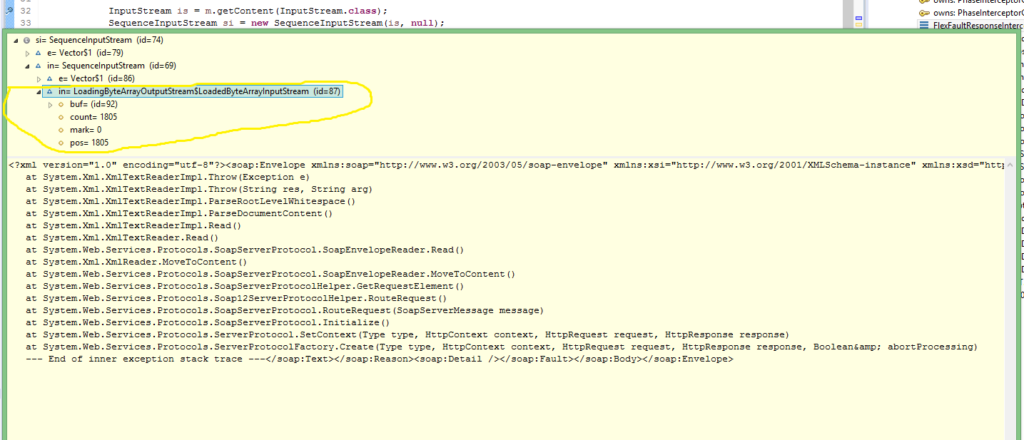
The Sequence Input Stream class extends the Input Stream class and implements the Closeable and Auto Closeable interfaces. The general explanation of input sequence flow class in Java is given below: public class Sequence Input Stream extends Input Stream implements Closeable, AutoCloseable The SequenceInputStream class was added in Java version 1.0. It is available in the java.io.Sequence Input Stream package.
The constructor of the class Sequence Input Stream in Java
The Sequence Input Stream class defines two constructors that are different from any other Input Stream. They are as follows: 1. Sequence Input Stream(Input Stream s1, Input Stream s2) : This constructor creates a new Sequence Input Stream object that takes two Input Stream objects as arguments and combines them into a single input stream. It reads the data (in bytes) in order, first s1, then s2. A z. B. To read the data from file1 and file2, we can proceed as follows: File Input Stream file1 = new File Input Stream(text1.txt) ; File Input Stream file2 = new File Input Stream(text2.txt) ; //concatenate file1 and file2 to file3. Sequence Input Stream file3 = new Sequence Input Stream(file1, file2) ; Take a look at the following figure to understand it better. 2. Sequence Input Stream(Enumeration e) : This constructor creates a new Sequence Input Stream which takes an enum Input Streams as argument. It reads data from an enumeration whose type is Input Stream.
SequenceInputStream methods in Java
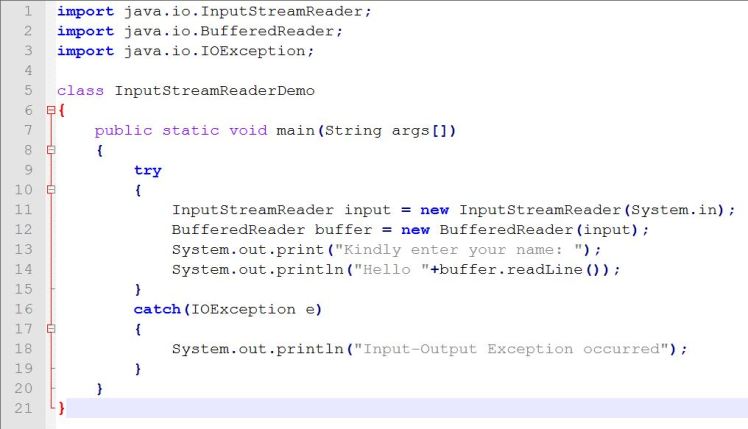
In addition to the methods inherited from the Input Stream class, the Sequence Input Stream class also defines some useful methods in Java. They are as follows:
Methods | Description |
1. int available() : | This method returns the actual number of bytes that can be read (or skipped) from the current basic input stream without blocking the next method call. |
2. void close() : | This method closes the input thread and releases all system resources associated with the thread. |
3. int read() : | This method reads the next data byte in the basic input stream. |
4. int read(byte[ ] b, int n, int m) : | This method reads up to m bytes of data from the underlying input stream into a byte array. |
All these methods throw an exception called IOException when an error occurs.
Examples of SequenceInputStream programs
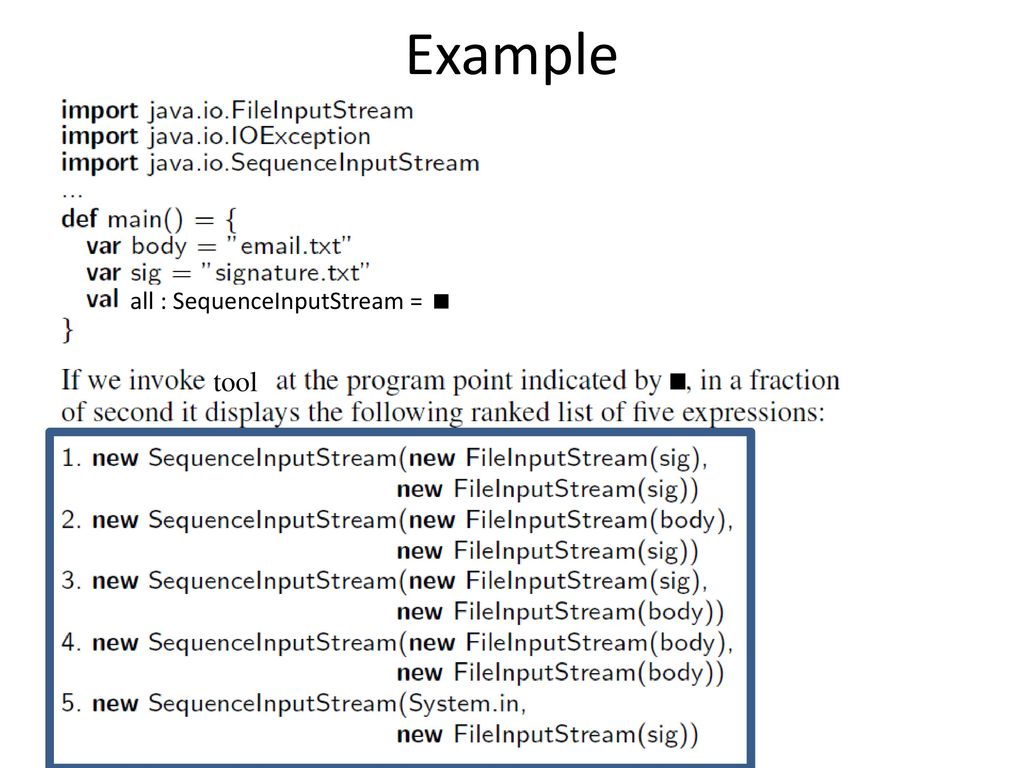
1. Let’s create a program that retrieves the data from two files file1 and file2 and prints them to the console. Take a look at the following source code. Source code of program 1 : package java Program ; import java.io.File Input Stream ; import java.io.IOException ; import java.io.Sequence Input Stream; public class Sequence Input StreamEx { public static void main(String[] args) throws IOException { FileInputStream file1 = new File Input Stream(D:\file1.txt); File Input Stream file2 = new File Input Stream(D:\file2.txt) ; //concatenate file1 and file2 to file3. Sequence Input Stream file3 = new Sequence Input Stream(file1, file2);int i;while((i = file3.read()) != -1) {System.out.print((char)i);}file3.close();file2.close();file1.close();} In this program, we assume you have two files: file1.txt and file2.txt, which contain the following data:
- File 1: Welcome to the world of Java programming.
- file2 : Here is an example of a Java Sequence Input Stream class.
After running the above program, the following result is displayed: Exit: Welcome to the world of Java programming. Here is an example of a Java SequenceInputStream class. This program is a simple example of the process of concatenating two files into one. In this program, we created two FileInputStream class objects for the files file1.txt and file2.txt.
Two reference variables of the FileInputStream object file1 and file2 are passed as arguments to the SequenceInputStream constructor to create a single object input stream file. File 3 now contains a combination of file 1 and file 2 and prints the data from file 3 to the console after concatenation. 2. Let’s take the example of a program in which we want to merge, cache and display data from two independent files. Program source code 2 : package javaProgram; import java.io.Buffered Input Stream; import java.io.Buffered Output Stream; import java.io.File Input
Stream; import java.io.IOException; import java.io.Sequence Input Stream; public class Sequence Input StreamEx { public static void main(String[] args) throws IOException { FileInputStream file1 = new File Input Stream(D:\file1.txt); File Input Stream file2 = new File Input Stream(D:\file2.txt) ; //concatenate file1 and file2 to file3. Sequence Input Stream file3 = new Sequence Input Stream(file1, file2) ; // Now create buffered input and output stream objects. Buffered Input Stream bis = new Buffered Input Stream(file3); Buffered Output Stream bos = new Buffered Output Stream(System.out) Read and write to the end of the buffer.int i;while((i = bis.read()) != -1) {bos.write((char)i);}bis.close();bos.close();file1.close();file2.close();} In this program, we assume you have the following content:
- File 1: Hello!
- File 2: Welcome to the world of Java programming.
After running this program, get the following output on the console. Exit: Hello! Welcome to the world of Java programming. This program shows the whole process of concatenating, buffering and displaying the data of two independent files correctly. 3. Let’s take the example of a program that reads data from two files file1.txt and file2.txt and writes them to another file file fileout.txt.
Take a look at the source code to understand it better. Program source code 3 : java Program package; import java.io.Buffered Input Stream; import java.io.Buffered Output Stream; import java.io.File Input Stream; import java.io.FileOutputStream; import java.io.IOException; import java.io.Sequence Input Stream; public class Sequence Input StreamEx { public static void main(String[] args) throws IOException { File Input Stream file1 = new File Input Stream(D:\file1.txt); File Input Stream file2 = new File Input Stream(D:\file2.txt); File Output Stream fos = new File Output Stream(D:\fileout.txt) ; File Sequence Input Stream3 = new Sequence Input Stream(file1,file2)
Now create buffered input and output stream objects. Buffered Input Stream bis = new Buffered Input Stream(file3); Buffered Output Stream bos = new Buffered Output Stream(fos) Read and write to the end of the buffer. int i; while((i = bis.read()) != -1){ bos.write((char)i); } bis.close(); bos.close(); fos.close(); file1.close(); file2.close(); file3.close(); System.out.println(Write succeeded…); } } Exit: Successfully written… fileout.txt : Hello! Welcome to the world of Java programming. 4. Let’s create a program to read data from files using enumeration.
If you need to read data from more than two files, you can use enumeration. An enumeration object can be created by calling the elements() method of the vector class. Check the source code. Program source code 4 : package javaProgram ; import java.io. File Input Stream ; import java.io.IOException ; import java.io. Sequence Input Stream ; import java.util.Enumeration ; import java.util.Vector; public class Sequence Input Stream Ex { public static void main(String[] args) throws IOException { File Input Stream file1 = new File Input Stream(D:\file1.txt); File Input Stream file2 = new File Input Stream(D:\file2.txt); File Input Stream file3 = new File Input Stream(D:\file3.txt); File Input Stream file4 = new File Input Stream(D:\file4.txt) Create a
Vector class object for all threads. Create an enumeration object by calling the elements method of the vector class. Enumeration and = v.elements() ; // Pass the enumeration object to the constructor of the SequenceInputStream class. In this program, we assume that you have the following data in four files.
- File 1: Welcome.
- file2: in Java
- file3 : Programming
- file4 : The world.
After running the program, you will get the following result: Exit: Welcome to the world of Java programming. I hope this tutorial has covered all the important points of the Sequence Input Stream class in Java in detail with example programs. I hope you understood all these basic points of the current order of entry.
Thanks for reading!!!The java.io package provides classes that deal with reading and writing to and from files, and manipulating those files. These classes can be used to read or write to a file or other input stream in several different ways. The java.io.ByteArrayInputStream and java.io.Input Stream classes allow you to read and write data to or from files in binary format.
The java.io.InputStreamReader and java.io.OutputStreamWriter classes allow you to read or write text to or from a file. The java.io.FileInputStream and java.io.FileOutputStream classes allow you to read or write to files in textual format. The java.io.File class provides a means of creating a new file. Read more about sequenceinputstream class is used to read data from and let us know what you think.
Frequently Asked Questions
What is SequenceInputStream in Java?
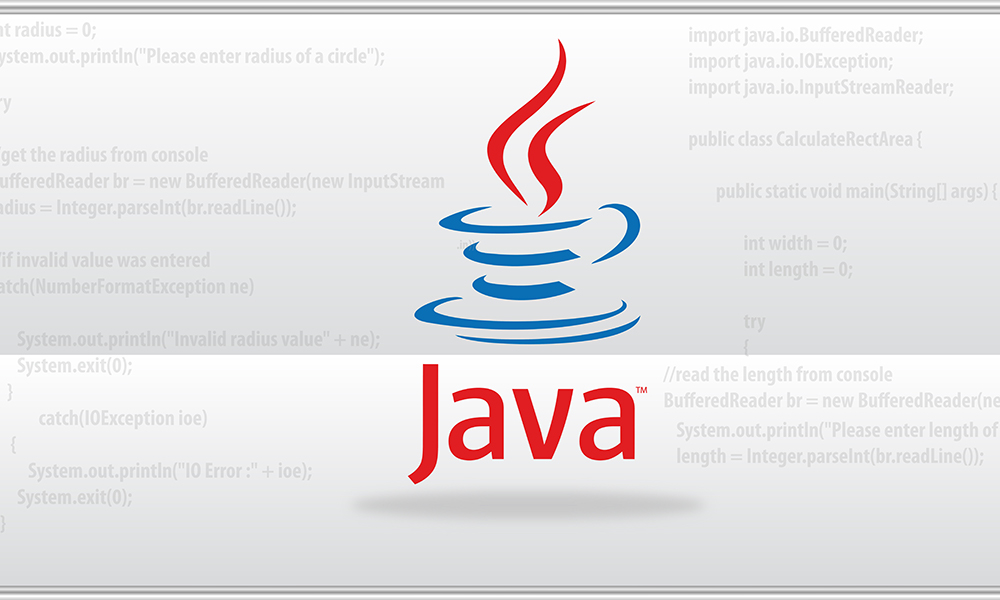
A SequenceInputStream is an input stream that supports sequential reads. In this article, we demonstrate how to use the SequenceInputStream class to read sequence data and provide random access to the data.
How can we access multiple files by a single stream?
SequenceInputStream is a type of InputStream that provides a chained method for reading multiple files from a single stream using a byte array as buffer. In this blog post, we will be discussing the java.io classes that support adding data to a sequence in sequential form.
We will also be discussing how to read multiple streams from a single input file. This information will be useful to those who are learning about working with streams and want to learn how to access multiple streams by a single input stream. The file input stream in java.io.FileInputStream and java.io.InputStream are both sequences of lines of text.
Both streams can only read one line at a time from a file and will read the entire file or all lines in the file into memory before reading the next line. In addition, the input methods sections discusses ways to skip over lines of text from a file, or
What are the two input stream classes?
InputStream and OutputStream are 2 basic interfaces that are used by the standard java.io package. The InputStream interface is used to read data from a source while the OutputStream interface is used to write data to a destination. Both are contained in the java.io package.
At this point you should have a working Java app that handles appending to a list, and has a few methods that pop a list item at random and then pop an item off of an array. In the process of populating that array we’re going to be using the two input stream classes that we’ve been using in this tutorial, namely RandomAccessFileInputStream and RandomAccessDirectoryIterator.